Welcome to a guide on two of SwiftUI's most important property wrappers: @State and @Binding.…
Master SwiftUI’s @State – A Step-by-Step Guide to Dynamic UIs
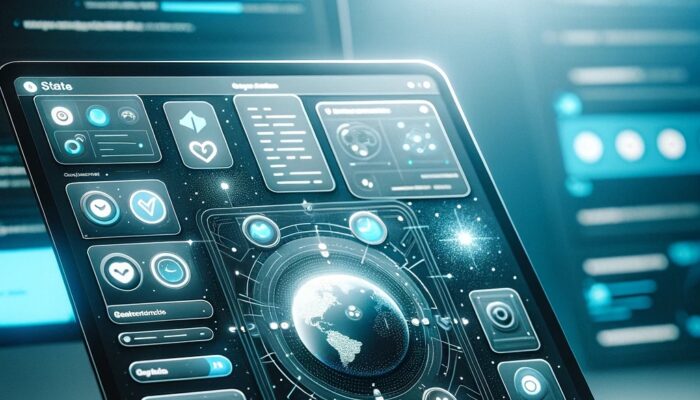
Dive into the exciting world of SwiftUI with our beginner-friendly tutorial! In this post, we’ll explore the @State
property wrapper, a fundamental concept in SwiftUI for managing local state within your views. With insights from WWDC 2023, this guide will help you understand and implement @State
in your SwiftUI apps using the latest techniques and best practices.
Step 1: Understanding @State
@State
is a property wrapper in SwiftUI used for creating local, mutable state within a view. When a @State
property changes, SwiftUI automatically re-renders the view to reflect the new state. This makes it perfect for handling user interactions and dynamic content in your app.
Step 2: Setting Up Your SwiftUI Project
First, make sure you have Xcode installed and create a new SwiftUI project. We’ll build a simple app where users can toggle a light bulb on and off.
import SwiftUI
Step 3: Creating a Light Bulb View with @State
We’ll now create a view with a light bulb image that users can tap to turn on and off. The light bulb’s state (on or off) will be managed by a @State
variable.
struct LightBulbView: View {
@State private var isLightOn = false
var body: some View {
Image(systemName: isLightOn ? "lightbulb.fill" : "lightbulb")
.resizable()
.frame(width: 100, height: 100)
.foregroundColor(isLightOn ? .yellow : .gray)
.onTapGesture {
isLightOn.toggle()
}
}
}
Step 4: Previewing Your Light Bulb
SwiftUI provides a powerful preview tool to see your UI in action. Here’s how you can preview the LightBulbView
.
#Preview {
LightBulbView()
}
Step 5: Exploring @State Further
@State
is particularly useful for simple local data that belongs to a single view. It’s important to remember that @State
should be private to maintain the view’s source of truth.
Conclusion
Congratulations! You’ve just created a dynamic light bulb toggle using @State
in SwiftUI. This tutorial showcased how @State
allows for interactive and responsive UI components, an essential skill in modern iOS app development. Keep experimenting with @State
to build more interactive features and enhance your SwiftUI apps.
Stay tuned for more tutorials that dive deeper into SwiftUI’s capabilities.
Happy coding! 💡👩💻👨💻