The SwiftUI @Environment property wrapper allows views to access shared data and respond to changes…
How to use @Bindable in SwiftUI
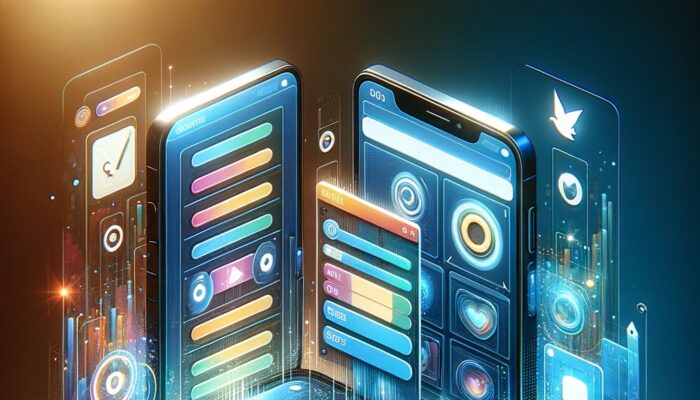
In this beginner-friendly tutorial, we’ll explore the use of SwiftUI’s @Bindable
property wrapper, a tool that enhances data binding in SwiftUI apps.
Step 1: Understanding @Bindable
@Bindable
creates bindings to properties of observable objects, allowing for direct UI interactions with the data model.
Step 2: Setting Up the Observable Object
Define a Book
class with properties for title and availability:
import SwiftUI
@Observable
class Book {
var title = "Sample Book Title"
var isAvailable = true
}
Step 3: Creating the Book Editing View
Build a BookEditView
that uses @Bindable
for real-time editing:
struct BookEditView: View {
@Bindable var book: Book
var body: some View {
Form {
TextField("Title", text: $book.title)
Toggle("Book is available", isOn: $book.isAvailable)
}
}
}
Step 4: Implementing in ContentView
In ContentView
, instantiate Book
and pass it to BookEditView. Also add Text with book.title and book.isAvailable .
struct ContentView: View {
var book = Book()
var body: some View {
BookEditView(book: book)
Text(book.title)
Text(book.isAvailable ? "Available" : "Unavailable")
}
}
#Preview {
ContentView()
}
Step 5: Exploring @Bindable Further
Experiment with @Bindable
by adding more properties to Book
and updating BookEditView
accordingly.
Conclusion
@Bindable
in SwiftUI simplifies creating interactive forms and UIs directly linked with your data model. Practice by adding more features and observe the ease of UI updates with @Bindable
.
Apple Documentation: @Bindable
Learn more about @State and @Binding
Happy SwiftUI coding! 🌟👩💻👨💻