The SwiftUI @Environment property wrapper allows views to access shared data and respond to changes…
SwiftUI’s @State and @Binding differences
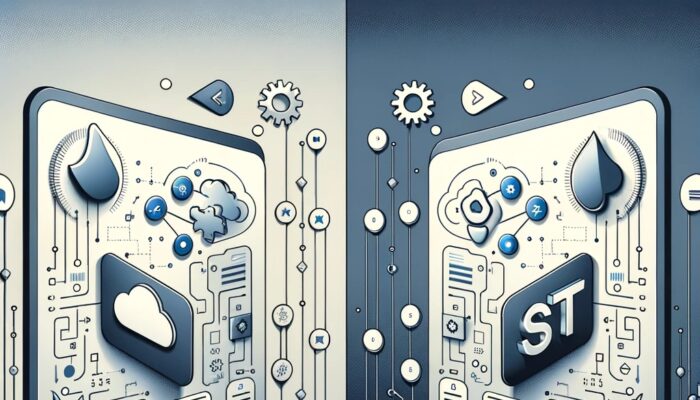
Welcome to a guide on two of SwiftUI’s most important property wrappers: @State
and @Binding
. These two tools are essential for managing data flow in SwiftUI apps. In this post, we’ll explore their differences through simple explanations and code examples, making it easy for everyone to grasp these concepts.
Understanding @State
@State
is used for data that is owned by the view. It’s your view’s private data storage.
Simple Example: Imagine you have a view with a counter that increases every time a button is tapped. Here’s how you might use @State
:
struct CounterView: View {
@State private var count = 0
var body: some View {
VStack {
Text("Tap Count: \(count)")
Button("Tap Me!") {
count += 1
}
}
}
}
In this example, count
is a @State
variable that keeps track of the tap count. When the button is tapped, count
is updated, and the view automatically refreshes to show the new count.
Understanding @Binding
@Binding
creates a two-way connection between the view and data that is managed elsewhere.
Simple Example: Now, imagine you have a parent view that controls whether a child view is visible or not. Here’s how @Binding
can be used:
struct ParentView: View {
@State private var isChildVisible = false
var body: some View {
VStack {
Toggle("Show Child View", isOn: $isChildVisible)
if isChildVisible {
ChildView(isVisible: $isChildVisible)
}
}
}
}
struct ChildView: View {
@Binding var isVisible: Bool
var body: some View {
Button("Hide Me") {
isVisible = false
}
}
}
In ParentView
, isChildVisible
is a @State
variable. ChildView
receives this state as a @Binding
, which means ChildView
can update isChildVisible
, and these changes are reflected in ParentView
.
Key Differences
- @State is for internal data in a view – like a private notebook.
- @Binding is for sharing data between views – like a walkie-talkie for data communication.
Let’s break down the main differences between @Binding
and @State
in SwiftUI with simple explanations:
@State
- Ownership:
@State
is used when your view owns the data. Think of@State
as a personal notebook that belongs to the view. The view writes down its data in this notebook, and whenever the data changes, the view knows it needs to update itself. - Source of Truth: It’s the primary source of truth for data within the view. If your view is creating and managing the data it displays, you use
@State
. - Local to View:
@State
is generally private and local to the view. It’s not shared with other views directly.
@Binding
- Shared Data:
@Binding
comes into play when you need to create a two-way connection between the view and data that is owned by another part of your app. Think of@Binding
as a walkie-talkie that your view uses to communicate with the main data source. - Not the Source of Truth: It does not own the data. Instead, it’s like a reference or a link to the data owned by another view or a parent view.
- Used for Passing Data: You use
@Binding
when you want to pass data around between different views while keeping them in sync.
Conclusion
@State
and @Binding
are crucial for managing data in SwiftUI. @State
is used for data owned by a single view, while @Binding
is for sharing data between views. Understanding these concepts is key to building dynamic and responsive SwiftUI apps.
Read more about @Binding
Read more About @State
Experiment with these examples and try creating your own scenarios to better understand how @State
and @Binding
work in SwiftUI.