The SwiftUI @Environment property wrapper allows views to access shared data and respond to changes…
SwiftUI @Observable Feature: Simplified Data Management – WWDC 23 Update
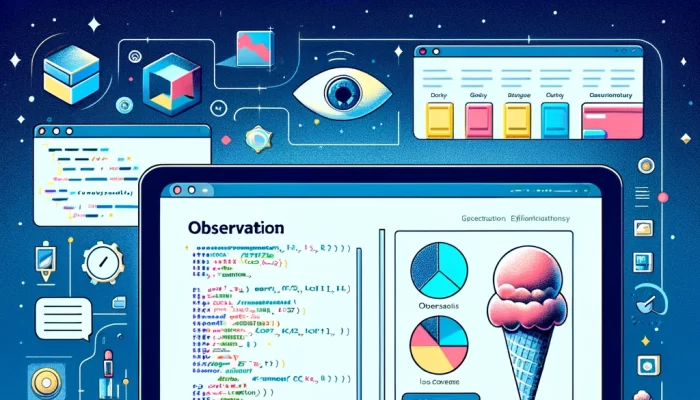
Welcome to a fun and easy introduction to app development with SwiftUI! This tutorial will guide you through creating an Ice Cream Flavors List app using SwiftUI’s @Observable
feature, introduced at WWDC 23. Let’s dive into each step, explaining how @Observable
works and how you can use it to create dynamic apps.
Step 1: Grasping the Concept of @Observable
The @Observable
feature in SwiftUI is a game-changer. It’s a tool that allows your app to automatically update its display whenever the data it’s showing changes. Think of it as an alert system: whenever you add a new ice cream flavor to your list, @Observable
tells the app to show this new flavor right away.
Step 2: Setting Up the Ice Cream Store
First, let’s set up a store for our ice cream flavors. We’ll create a class called IceCreamStore
and use the @Observable
attribute. This class will hold a list of flavors and a function to add new flavors.
import SwiftUI
@Observable
class IceCreamStore {
var flavors: [String] = ["Vanilla", "Chocolate", "Strawberry"]
func addFlavor(newFlavor: String) {
if !newFlavor.isEmpty {
flavors.append(newFlavor)
}
}
}
This code creates a store with three initial flavors. The addFlavor
function lets us add more flavors to this list.
Step 3: Designing the Ice Cream List View
Now, let’s create the part of our app that users will see and interact with. This is called a view in SwiftUI. In our view, we’ll show the list of flavors and provide a way to add new flavors.
struct IceCreamListView: View {
var store = IceCreamStore()
@State private var newFlavor = ""
var body: some View {
VStack {
List(store.flavors, id: \.self) { flavor in
Text(flavor)
}
VStack(spacing: 20) {
TextField("Enter new flavor", text: $newFlavor)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
Button("Add") {
store.addFlavor(newFlavor: newFlavor)
newFlavor = ""
}
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
Spacer()
}
.padding()
}
}
}
In this view, we display each flavor in a list. Below the list, there’s a text field to enter a new flavor and a button to add it to the list.
Step 4: Previewing and Testing
SwiftUI lets us preview our app in real-time. You can see how your app looks and how it responds to interactions using the #Preview
section.
#Preview {
IceCreamListView()
}
Step 5: Adding New Flavors and Seeing @Observable in Action
When you run your app and type a new flavor in the text field, then press “Add”, the addFlavor
function in IceCreamStore
is called. This function adds the new flavor to the flavors
array. Because we’ve marked IceCreamStore
with @Observable
, the app knows it needs to update the list to include the new flavor you just added.
Conclusion
You’ve just created an Ice Cream Flavors List app using SwiftUI’s new @Observable
feature! This tutorial provided a step-by-step guide on using @Observable
to make dynamic apps that respond to data changes.
As you continue your coding journey, remember that experimenting and building projects is a great way to learn. Try adding new features to your app and explore the possibilities with SwiftUI! Happy coding! 🍦👨💻👩💻