The SwiftUI @Environment property wrapper allows views to access shared data and respond to changes…
SwiftUI @Binding Tutorial
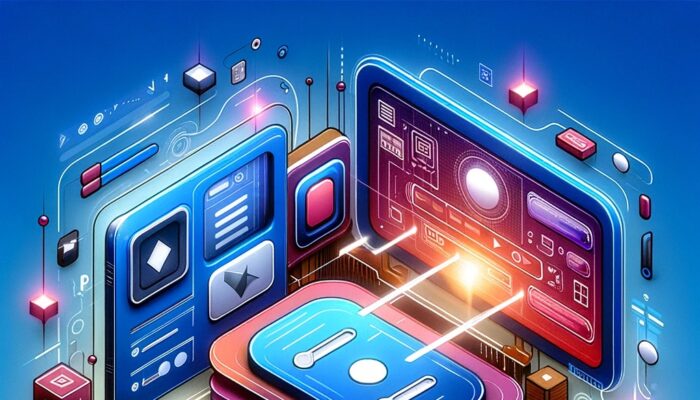
Embark on your SwiftUI journey with this beginner-friendly tutorial focused on mastering the @Binding
property wrapper! This guide is tailored for newcomers eager to learn how to create responsive and interactive user interfaces in SwiftUI.
Step 1: Understanding @Binding
@Binding
is a SwiftUI property wrapper that creates a two-way binding between a view and its data. When you use @Binding
, you can ensure that when the data changes, the view updates to reflect those changes, and vice versa. It’s crucial for interactive UI elements like sliders, text fields, and toggles.
Step 2: Setting Up Your SwiftUI Project
Ensure you have the latest version of Xcode installed and start a new SwiftUI project. We’ll create a simple app where users can adjust a slider to change the size of an image.
import SwiftUI
Step 3: Building a Slider and Image View with @Binding
Let’s create a view containing a slider and an image. The slider will adjust the size of the image. We’ll use @Binding
to link the slider’s value to the image’s size.
First, create a separate view for the slider:
struct SizeSliderView: View {
@Binding var imageSize: CGFloat
var body: some View {
Slider(value: $imageSize, in: 50...150)
}
}
In SizeSliderView
, imageSize
is a @Binding
variable. It’s connected to a parent view that owns the data.
Now, create the main view:
struct ContentView: View {
@State private var imageSize: CGFloat = 100
var body: some View {
VStack {
Image(systemName: "photo")
.resizable()
.frame(width: imageSize, height: imageSize)
SizeSliderView(imageSize: $imageSize)
}
}
}
In ContentView
, imageSize
is a @State
variable. It’s the source of truth for the size of the image. The SizeSliderView
receives this state as a binding.
Step 4: Previewing Your Interactive UI
Utilize SwiftUI’s preview feature to see how your UI behaves in real time:
#Preview {
ContentView()
}
Step 5: Experimenting with @Binding
Try changing the range of the slider or the initial imageSize
state. Observe how the @Binding
ensures the slider and image size stay in sync.
Conclusion
You’ve successfully created an interactive UI using @Binding
in SwiftUI! This tutorial showcased the power of @Binding
in creating responsive UI elements that react to user input. Explore further by implementing @Binding
in different scenarios and discover the versatility it offers in SwiftUI app development.
Learn more about @State and @Bindable
Keep learning and experimenting, and stay updated with the latest SwiftUI features and best practices.
Happy SwiftUI coding! 🎨👩💻👨💻