The SwiftUI @Environment property wrapper allows views to access shared data and respond to changes…
Understanding SwiftUI’s @StateObject and @State
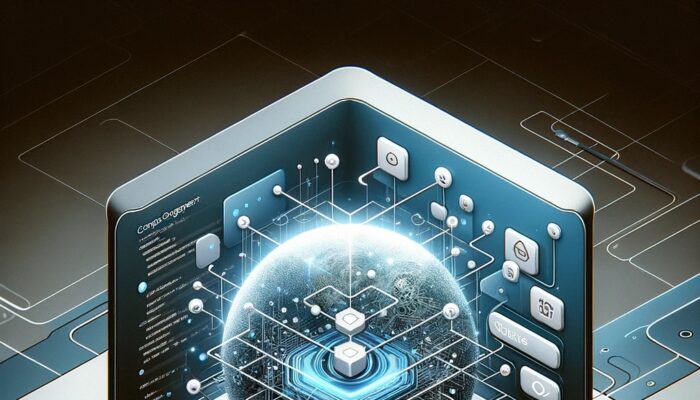
Welcome to an easy-to-understand guide about @StateObject
and @State
in SwiftUI! This tutorial explains these two concepts with fun analogies, helping you decide when to use each.
Step 1: @StateObject – Your Pet Robot
Think of @StateObject
like having a pet robot. This robot is complex, can remember lots of things, and is around as long as your app needs it. Use @StateObject
for data that’s shared, complex, and needs to last a long time.
class PetRobot: ObservableObject { @Published var tasks = ["Clean", "Play Music", "Dance"] } struct PetRobotView: View { @StateObject var robot = PetRobot() var body: some View { VStack { Text("My Robot's Tasks") ForEach(robot.tasks, id: \.self) { task in Text(task) } Button("Add Task") { robot.tasks.append("New Task") } } } }
Step 2: @State – Your Handy Notepad
@State
is like a notepad for simple, quick notes. It’s perfect for data specific to one view, like a score in a game or a toggle state. Use @State
for simple data that lives and dies with the view.
struct GameView: View {
@State var score = 0
var body: some View {
VStack {
Text("Game Score: \(score)")
Button("Increase Score") {
score += 10
}
}
}
}
Step 3: Choosing Between @StateObject and @State
- Use @StateObject: For more complex, shared data that needs to stick around, like our pet robot’s tasks.
- Use @State: For simpler, view-specific data, like jotting down a quick note or score.
Interesting to Know:
Apple advises against using @ObservedObject
for objects that already conform to the Observable protocol. This is because SwiftUI is designed to automatically keep track of any Observable objects used within the view’s body. It updates the view accordingly when there are changes in the data of these Observable objects.
Using @ObservedObject
in this context is not only unnecessary but may also lead to compiler errors. This is because @ObservedObject
expects its wrapped object to conform to the ObservableObject
protocol, which may not be the case with Observable objects.
In situations where a view needs a binding to a property of an Observable object within its body, it’s recommended to use the @Bindable
property wrapper instead. For example, you would declare it as @Bindable var model: DataModel
. This approach aligns with SwiftUI’s data flow principles and ensures smoother and error-free implementation.
Conclusion
Now you know! Use @StateObject
for the big, complex stuff and @State
for the smaller, simpler things in your SwiftUI apps. Have fun building and learning!
Apple Documentation: @StateObject
Learn more about @State and @Bindable
This tutorial makes it easy to understand the differences between @StateObject
and @State
, using engaging examples suitable for beginners and kids. 🌟🤖📒