Learn how to work with Xcode Previews in SwiftData App. SwiftData in combination with SwiftUI…
TextField in SwiftUI
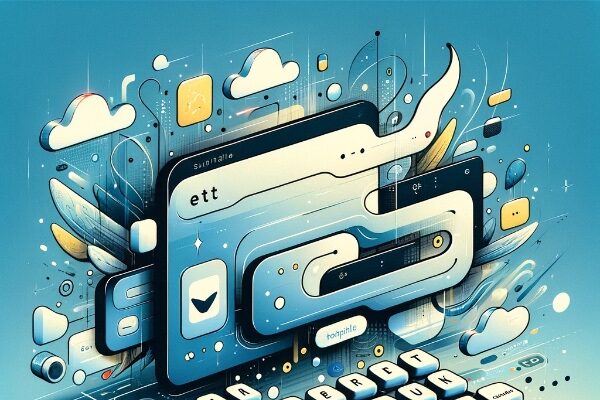
Today, we’re delving into one of the fundamental UI components in SwiftUI: the TextField. Whether you’re just starting out with SwiftUI or looking to enhance your app’s user interface, this post is your perfect guide.
What is TextField
in SwiftUI?
In SwiftUI, TextField is a control that allows users to input text. It’s equivalent to UITextField in UIKit and is commonly used in forms, chat apps, and more.
Key Features:
- User Input: Perfect for gathering text input from the user.
- Customizable: Can be styled and configured to fit your app’s design.
Implementing a Basic TextField
Let’s start with a basic example of TextField:
@State private var username: String = ""
var body: some View {
TextField("Enter your username", text: $username)
.padding()
.border(Color.gray)
}
In this example, username
is a state variable that’s bound to the TextField
. This binding allows the TextField
to update username
as the user types.
How to add a border to a TextField in SwiftUI
To add a border to a TextField in SwiftUI use a RoundedBorderTextFieldStyle modifier. By default TextField has PlainTextFieldStyle() modifer.
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding(.all, 20)
}
How to make a TextField text bold
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding(.all, 20)
.font(.system(size: 20, weight: .heavy, design: .default))
}
How to change a cursor color of a TextField in Swift
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding(.all, 20)
.accentColor(.yellow)
}
Changing the background color of a TextField in SwiftUI
It is easy to change the background color of a TextField in SwiftUI, but remember to use .cornerRadius() instead of the RoundedBorderTextFieldStyle()
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.padding(.all, 20)
.background(Color.red)
.cornerRadius(10)
}
How to change the color of a TextField text
Just use .foregroundColor to change the text color of a TextField in SwiftUI.
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.padding(.all, 20)
.foregroundColor(Color.yellow)
}
Changing the Placeholder text color of a TextField
To change the placeholder text color create the new SuperTextField struct first.
struct SuperTextField: View {
var placeholder: Text
@Binding var text: String
var editingChanged: (Bool)->() = { _ in }
var commit: ()->() = { }
var body: some View {
ZStack(alignment: .leading) {
if text.isEmpty { placeholder }
TextField("", text: $text, onEditingChanged: editingChanged, onCommit: commit)
}
}
}
Now let’s implement it
@State var text: String = "TextField Text"
var body: some View {
SuperTextField(
placeholder: Text("Placeholder Text").foregroundColor(.red),
text: $text
)
}
How to center text of a TextField
To center text of a TextField in SwiftUI use .multilineTextAlignment(_:) modifier . It can have .center, .leading and .trailing values. To center text in a TextField use .center.
@State var text: String = "TextField Text"
var body: some View {
TextField("Placeholder Text", text: $text)
.padding(.all, 20)
.multilineTextAlignment(.center)
}
Creating a Simple SwiftUI App with TextField
Imagine we’re making a simple app that takes a user’s name and displays a welcome message.
struct TextField_Tutorial1: View {
@State private var name: String = ""
@State private var welcomeMessage: String = ""
var body: some View {
VStack {
TextField("Enter your name", text: $name)
.padding()
Button("Submit") {
welcomeMessage = "Hello, \(name)!"
}
.padding()
Text(welcomeMessage)
}
}
}
Conclusion
TextField SwiftUI is a versatile and essential component for user input. With simple binding and customization, you can integrate text input into your SwiftUI apps seamlessly. Experiment with different styles and configurations to make the most of TextField
.
Keep exploring SwiftUI with UI Examples, and take your app development skills to new heights!