In SwiftUI development, a common question arises: Should you use structs or classes for your…
Swift Bool value type: A full Guide to True or False
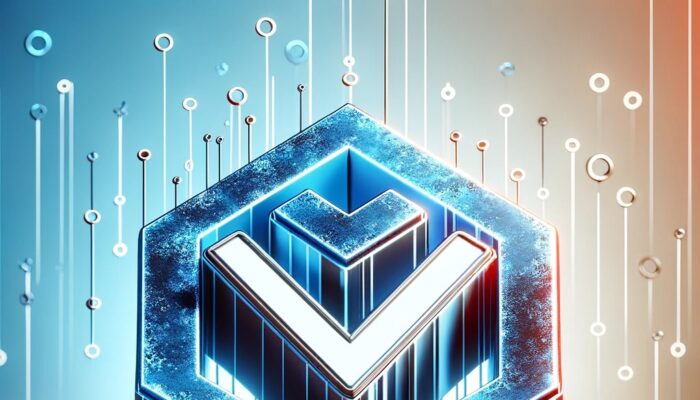
Let’s discover Swift Bool Value type.
What is Bool
in Swift?
In Swift, Bool
is a value type that represents boolean values β it can only be either true
or false
. Understanding Bool
is essential for controlling the flow of your programs with conditions and loops.
Key Features of Bool
:
- Binary Nature: A
Bool
value is eithertrue
orfalse
, nothing else. - Control Flow: Itβs commonly used in conditional statements like
if
,while
, andfor
.
Simple Bool
Examples
Basic Usage
Let’s start with a simple Bool
declaration:
var isCompleted: Bool = false
isCompleted = true // Updating the value
Using Bool
in Conditions
Bool
shines in conditional statements:
var hasPassedTest = true
if hasPassedTest {
print("Congratulations! You've passed the test.")
} else {
print("Sorry, you didn't pass. Try again!")
}
Toggling a Bool
Swift provides a convenient way to toggle a boolean value:
var isLightOn = false
isLightOn.toggle() // Now isLightOn is true
Interesting Bool
Usage Examples
Interactive Game Example
In a simple game, you might use a Bool
to check if a player can access a level:
var hasKey = true
var levelUnlocked = false
if hasKey {
levelUnlocked = true
print("Level unlocked! Get ready to play.")
}
User Authentication
In an app, you could use a Bool
to manage user login status:
var isLoggedIn = false
// After successful login
isLoggedIn = true
if isLoggedIn {
print("Welcome to your dashboard!")
} else {
print("Please log in to continue.")
}
Bool
and Logical Operators
Bool
values become even more powerful when combined with logical operators like &&
(AND), ||
(OR), and !
(NOT).
Example with Logical Operators
let isWeekend = true
let isSunny = false
if isWeekend && isSunny {
print("It's a perfect day for a picnic!")
} else {
print("Let's find another activity.")
}
The Bool
type in Swift is a cornerstone for making decisions in your code. Its simplicity hides its power in controlling the flow of your programs. As you start your Swift journey, mastering Bool
will open doors to creating more complex and interactive applications.