Learn how to work with Xcode Previews in SwiftData App. SwiftData in combination with SwiftUI…
How to display sample SwiftData in SwiftUI with PreviewModifier
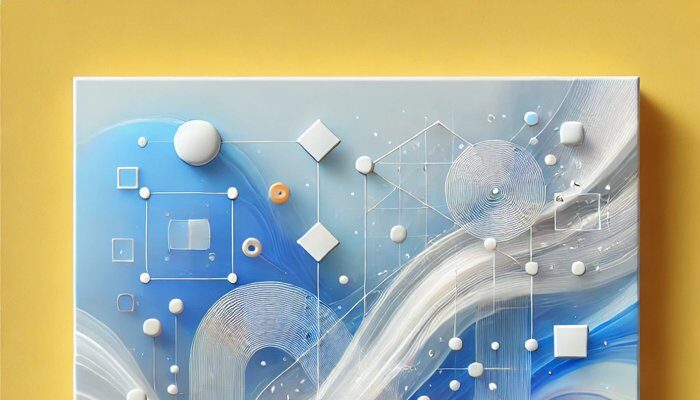
In this tutorial, we will explore how to display sample SwiftData in SwiftUI with PreviewModifier
Step 1: Define the Entity
First, we need to define a Task
entity that we will use to store our task data in SwiftData.
import Foundation
import SwiftData
@Model
class Task {
@Attribute(.unique) var id: UUID
var title: String?
var isCompleted: Bool
init(id: UUID = UUID(), title: String? = nil, isCompleted: Bool = false) {
self.id = id
self.title = title
self.isCompleted = isCompleted
}
}
In this code, we define the Task
model with an id
, title
, and isCompleted
property. The @Model
attribute is used to make this class a SwiftData model.
Step 2: Create the Task List View
Next, we’ll create a SwiftUI view that displays a list of tasks, each with a toggle for marking them as completed or not.
import SwiftUI
import SwiftData
struct TaskListView: View {
@Query(sort: \Task.title) private var tasks: [Task]
@Environment(\.modelContext) private var context
var body: some View {
List {
ForEach(tasks) { task in
HStack {
Text(task.title ?? "")
.font(.title2)
.foregroundColor(task.isCompleted ? .green : .black)
Spacer()
Toggle("", isOn: Bindable(task).isCompleted)
}
}
.onDelete(perform: deleteTask)
}
.task {
for task in sampleTasks {
context.insert(task)
}
}
.navigationTitle("Tasks")
}
private func updateTaskCompletion(_ task: Task, newValue: Bool) {
task.isCompleted = newValue
try? context.save()
}
private func deleteTask(at offsets: IndexSet) {
for index in offsets {
context.delete(tasks[index])
}
try? context.save()
}
}
In this view, each task can be marked as completed by toggling a switch, and we handle saving this state with SwiftData.
Step 3: Preview with SwiftData Context
To make the preview functional, we need to set up a SwiftData context. We’ll use the PreviewModifier
protocol to create a shared context that holds some sample data.
🔒 The remaining content of this article is only available on our Substack!
This Post Has 0 Comments