Learn how to work with Xcode Previews in SwiftData App. SwiftData in combination with SwiftUI…
GeometryReader in SwiftUI
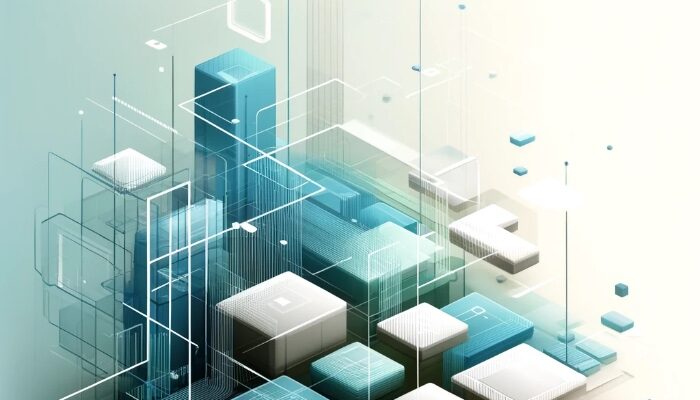
SwiftUI provides a flexible and powerful way to build user interfaces across all Apple platforms. GeometryReader allows you to read and manipulate the size and position of views dynamically. This tutorial will help you to use GeometryReader effectively.
What is GeometryReader?
GeometryReader
is a container view that defines its content as a function of its own size and coordinate space. This allows you to create adaptive layouts that respond to changes in view size and position.
Simple Example of GeometryReader
Let’s start with a basic example where we use GeometryReader
to create a view that changes its background color based on its size.
import SwiftUI
struct GeometryReaderExample: View {
var body: some View {
GeometryReader { geometry in
VStack {
Text("Width: \(geometry.size.width)")
Text("Height: \(geometry.size.height)")
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(geometry.size.width > 200 ? Color.blue : Color.red)
}
.frame(height: 200)
}
}
In this example, the background color of the VStack
changes based on the width of the GeometryReader
. If the width is greater than 200 points, the background color will be blue; otherwise, it will be red.
Using GeometryReader for Complex Layouts
Here is a more complex example that demonstrates how GeometryReader
can be used to create a responsive grid layout.
import SwiftUI
struct ResponsiveGridExample: View {
var body: some View {
GeometryReader { geometry in
let width = geometry.size.width
let columns = Int(width / 100)
let itemSize = width / CGFloat(columns)
VStack {
ForEach(0..<10) { row in
HStack {
ForEach(0..<columns, id: \.self) { column in
Rectangle()
.fill(Color.blue)
.frame(width: itemSize, height: itemSize)
}
}
}
}
}
.padding()
}
}
Explanation
- GeometryReader: This reads the size of the parent view.
- Calculating Columns and Item Size: We calculate the number of columns and the size of each item based on the width of the parent view.
- VStack and HStack: We use
VStack
andHStack
to create rows and columns. TheForEach
loop is used to generate the grid items dynamically. - Dynamic Range Fix: We use
id: \.self
in theForEach
loop to ensure each item is uniquely identified, which resolves the “Non-constant range” error.